Quick little tip for anyone who likes using habitica and also happen to use
Gnome Shell. I’ve been using Gnome-Pomodoro For
scheduling breaks and staying on task. I noticed the app also has support for
Custom actions. This can be really useful to update habitica habits when a pom
has been completed!
So I wrote a little script that will update your Pomodoro Habit
accordingly. It relies on Habitica CLI, so you’ll need to set up your account accordingly follow the instructions on the linked page.
Here’s the quick n’ dirty script:
|
|
You could also directly call the habitica program from the custom actions
window, but this will let you update/delete habits and still allow the script
to work since it references the habit name and not the habit number (which may change). It’s more of a “set and forget” solution!
Once you have the script, just add it as a custom action:
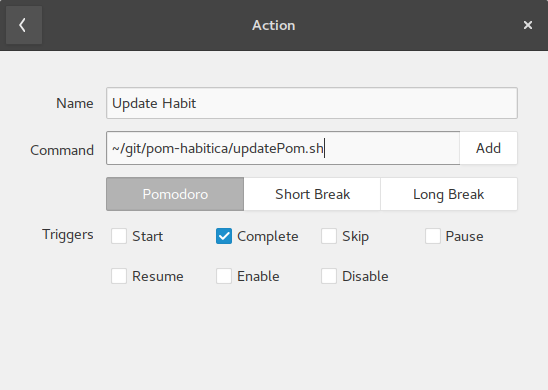
Possible Issues:
- Script only looks for two digit numbers in order to grab the correct habit to
update, You can change this accordingly. Check out this page for using regex with grep - Might break on updates to the habitica CLI